题目一
【问题描述】
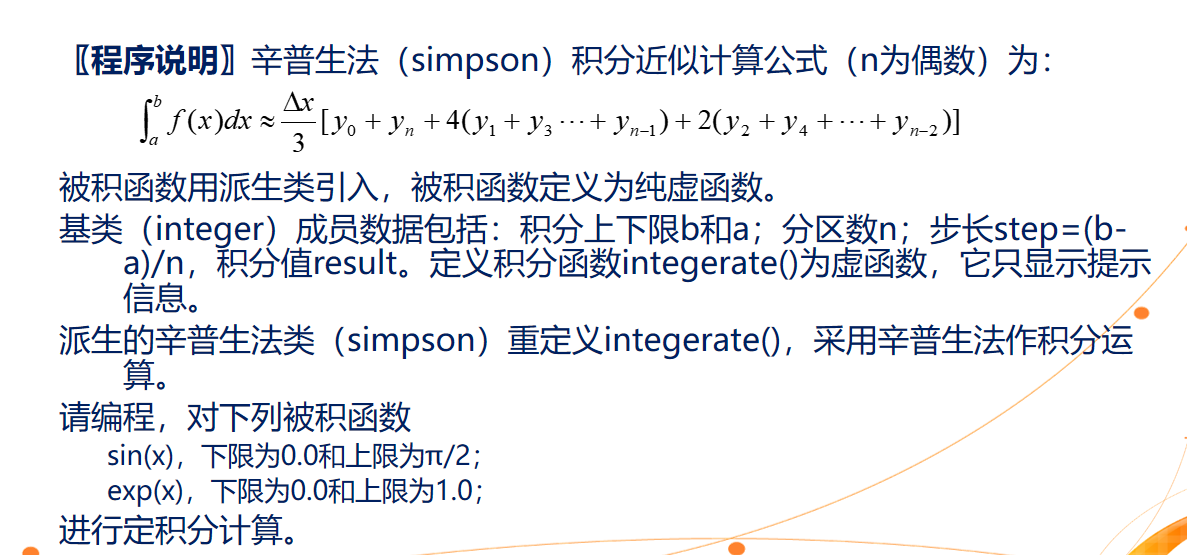
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106
| #include <iostream.h>
#include <math.h>
class Base{
public:
double result,a,b,step;
int n;
virtual double fun(double x) (1) ;
virtual void Integerate(){
cout<<"这里是积分函数"<<endl;
}
Base(double ra=0,double rb=0,int nn=2000){
a=ra; b=rb; n=nn; result=0;
}
void Print(){
cout.precision(15);
cout<<"积分值="<<result<<endl;
}
};
class Simpson:public Base{
public:
void Integerate(){
int i;
step=(b-a)/n;
result= (2) ;
for(i=1;i<n;i+=2) result= (3) ;
for(i=2;i<n;i+=2) result= (4) ;
result= (5) ;
}
Simpson(double ra,double rb,int nn): (6) {}
};
class sinS:public Simpson{
public:
sinS(double ra,double rb,int nn): (7) {}
double fun(double x){return sin(x);}
};
class expS: public sinS {
public:
expS(double ra,double rb,int nn): (8) {}
double fun(double x){return exp(x);}
};
void main(){
Base *bp;
sinS ss(0.0,3.1415926535/2.0,100);
bp= (9) ;
bp->Integerate();
bp->Print();
expS es(0.0,1.0,100);
bp= (10) ;
bp->Integerate();
bp->Print();
}
|
【答案】
1 2 3 4 5 6 7 8 9 10
| (1) 【 正确答案: 1=0】 (2)【 正确答案: fun(a)+fun(b)】 (3)【 正确答案: result+4fun(istep+a)】 (4)【 正确答案: result+2fun(istep+a)】 (5)【 正确答案: result*step/3】 (6)【 正确答案: Base(ra,rb,nn)】 (7)【 正确答案: Simpson(ra,rb,nn)】 (8)【 正确答案: sinS(ra,rb,nn)】 (9)【 正确答案: &ss】 (10)【 正确答案: &es】
|
题目二
【问题描述】
设公司有普通员工和经理两类人员。主函数已给出,编程设计普通员工类CommonWorker和经理类Manager,经理类除具有普通员工类的属性外。普通员工类的收入由基本工资和奖金构成,经理类的收入除基本工资和奖金外,还包括职务津贴。实现求两类员工工资的Pay函数。
【代码】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| #include <iostream> #include<iostream> using namespace std; class CommonWorker { public: virtual void Pay() { earning = wage + reward; cout << earning<<endl; } friend istream& operator>>(istream& inStream, CommonWorker& data) { inStream >> data.wage; inStream >> data.reward; return inStream; } protected: double wage; double reward; double earning; }; class Manager :public CommonWorker { public: virtual void Pay() { earning = wage + reward + place; cout << earning<<endl; } friend istream& operator>>(istream& instream, Manager& data) { instream >> data.wage; instream >> data.reward; instream >> data.place; return instream; } protected: double place; };
int main() { CommonWorker c1, * pc; Manager m1; cin >> c1 >> m1; pc = &c1; pc->Pay(); pc = &m1; pc->Pay(); return 0; }
|
题目三
【问题描述】
根据给出的程序片段编程,使程序正确运行。由基类Shape派生出圆类Circle(数据成员:半径)、正方形类Square(数据成员:边长)、三角形类Triangle(数据成员:三条边边长),3个派生类都有输入和显示信息函数Input、Output,计算面积的函数Area,计算周长的函数Perim。
【代码】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102
| #include <iostream> #include<iomanip> #include <cmath> using namespace std; const double PI = 3.14159;
class Shape { public: virtual double Area() const { return 0; } virtual double Perim() const { return 0; } virtual void Input() {} virtual void Output() { } static void SetFormat() { cout << setiosflags(ios::fixed) << setprecision(2); } protected: Shape(){} }; class Circle:public Shape { private: double mr; public: virtual void Input() { double r; cin >> r; this->mr = r; } Circle(double r = 0) { mr = r; } virtual void Output() { cout << PI * 2 * mr << "," << PI * mr * mr<<endl; } }; class Square :public Shape { private: double ma; public: Square(double a = 0, double b = 0) { ma = a; } virtual void Input() { double a; cin >> a; this->ma = a; } virtual void Output() { cout<<ma*4<<","<<ma*ma<<endl; } }; class Triangle :public Shape { private: double ma, mb, mc; public: Triangle(double a = 0, double b = 0, double c = 0) { ma = a; mb = b; mc = c; } virtual void Input() { double a, b, c; cin >> a >> b >> c; ma = a; mb = b; mc = c; } virtual void Output() { cout << ma + mb + mc << ","; double p, s; p = (ma + mb + mc) / 2; s = sqrt(p * (p - ma) * (p - mb) * (p - mc)); cout << s<<endl; } };
int main() { Circle circle; Square square; Triangle triangle; Shape* pt[3] = { &circle,&square,&triangle }; Shape::SetFormat(); for (int i = 0; i < 3; i++) pt[i]->Input(); for (int i = 0; i < 3; i++) pt[i]->Output(); return 0; }
|